Vue Js form validated to accept decimal numbers:In Vue.js, validating a form to accept decimal numbers involves several steps. First, you define a data property to store the input value. Then, you use the v-model directive to bind the input field to this property. To enforce decimal number validation, you can utilize the pattern attribute on the input field and specify a regular expression pattern like pattern=”[0-9]+(\.[0-9]+)?”.
This pattern allows for an optional decimal part. If the field is mandatory, you can include the required attribute. Finally, you can add a submit button and handle the form submission in a method that performs additional validation or processing. Combining these steps enables the creation of a Vue.js form that accepts and validates decimal numbers.
How can I validate an input field in Vuejs to accept decimal numbers?
This code snippet demonstrates a Vue.js form with an input field that is validated to accept decimal numbers. The input field is bound to the decimalValue
property using v-model
. The form has a submit event handler (submitForm
) that prevents the default form submission behavior.
Inside the submitForm
method, the input is validated using a regular expression. If the input is empty, an error message is set in the errors
object. If the input does not match the pattern for a decimal number, another error message is set. If the input is valid, the form can be submitted or other actions can be performed.
The errors are displayed below the input field using conditional rendering (v-if
). The is-invalid
class is added to the input field if there are errors, which can be used for styling purposes.
Overall, this code provides a basic validation mechanism for accepting decimal numbers in a Vue.js form.
Vue Js form Input field validated to accept decimal numbers:
<div id="app">
<h3>Vue Js form Input field validated to accept decimal numbers</h3>
<form @submit="submitForm">
<label for="decimalInput">Decimal Number:</label>
<input
type="text"
id="decimalInput"
v-model="decimalValue"
:class="{ 'is-invalid': errors.decimalInput }"
/>
<div v-if="errors.decimalInput" class="error">
{{ errors.decimalInput }}
</div>
<button type="submit">Submit</button>
</form>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
decimalValue: "",
errors: {},
};
},
methods: {
submitForm(event) {
event.preventDefault();
this.errors = {};
// Validate decimal input
if (!this.decimalValue) {
this.errors.decimalInput = "Decimal number is required.";
} else if (!/^\d+(\.\d+)?$/.test(this.decimalValue)) {
this.errors.decimalInput = "Invalid decimal number.";
} else {
// Decimal number is valid, submit the form or perform other actions
console.log("Decimal number:", this.decimalValue);
// Submit form or perform other actions here
}
},
},
});
app.mount("#app");
</script>
Output of Vue Js form Input field validated to accept decimal numbers
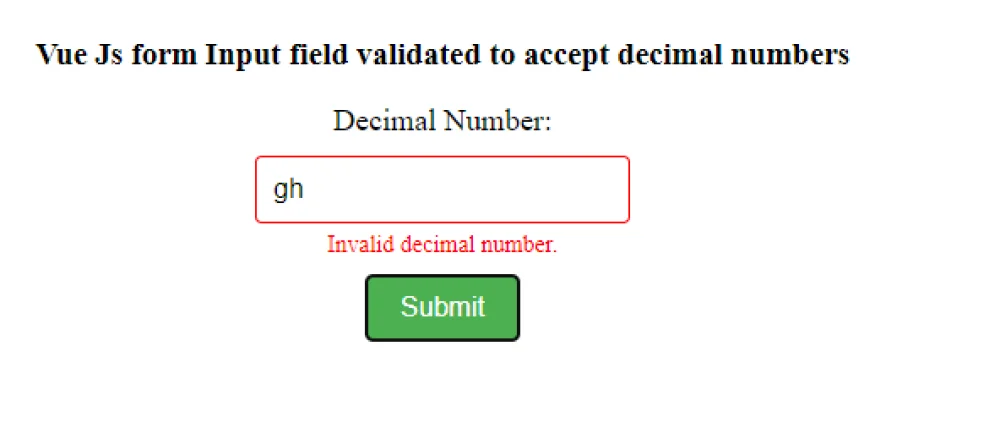